This post demonstrates adding validation on submitting register form.
Create GlobalKey<FormState> form key to validate the form.
final GlobalKey<FormState> _formKey = GlobalKey<FormState>();
Add validator to TextFormInput.
TextFormInput(
textEditingController: _confirmPasswordController,
labelText: 'Confirm Password (*)',
textInputType: TextInputType.text,isPass: true,
validator: (value) {
if (value == null || value.isEmpty) {
return 'This field is required';
} else if (value != _passwordController.text) {
return 'Passwords do not match';
}
return null;
},
),
Wrap the Column to Form Widget to handle the validator, and create button click listener function. Take a look at registerAccount(). Below is the full code.
import 'package:flutter/material.dart';
import 'package:gap/gap.dart';
import 'package:money_tracker/Screens/login_screen.dart';
import 'package:money_tracker/Utils/text_form_input.dart';
class RegisterScreen extends StatefulWidget {
const RegisterScreen({super.key});
@override
State<RegisterScreen> createState() => _RegisterScreenState();
}
class _RegisterScreenState extends State<RegisterScreen> {
final TextEditingController _emailController = TextEditingController();
final TextEditingController _passwordController = TextEditingController();
final TextEditingController _confirmPasswordController =
TextEditingController();
final GlobalKey<FormState> _formKey = GlobalKey<FormState>();
@override
void dispose() {
_emailController.dispose();
_passwordController.dispose();
_confirmPasswordController.dispose();
super.dispose();
}
void navigateToLoginScreen() {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => const LoginScreen()),
);
}
void registerAccount() {
final isValid = _formKey.currentState!.validate();
if (!isValid) {
return;
}
// proceed
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Container(
color: Colors.amber[50],
child: Center(
child: Padding(
padding: const EdgeInsets.all(20.0),
child: Container(
padding: const EdgeInsets.all(30),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(20),
boxShadow: const [
BoxShadow(
color: Color.fromARGB(255, 228, 228, 228),
spreadRadius: 4,
blurRadius: 4,
)
]),
width: 400,
child: Form(
key: _formKey,
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
width: 200,
height: 200,
child: Image.asset(
"assets/images/logo.png",
),
),
const Gap(20),
const Text(
'Create Account',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 25,
),
),
TextFormInput(
textEditingController: _emailController,
labelText: 'Email Address',
textInputType: TextInputType.emailAddress,
isRequired: true,
),
const Gap(10),
TextFormInput(
textEditingController: _passwordController,
labelText: 'Password',
textInputType: TextInputType.text,
isPass: true,
isRequired: true,
),
const Gap(10),
TextFormInput(
textEditingController: _confirmPasswordController,
labelText: 'Confirm Password (*)',
textInputType: TextInputType.text,
isPass: true,
validator: (value) {
if (value == null || value.isEmpty) {
return 'This field is required';
} else if (value != _passwordController.text) {
return 'Passwords do not match';
}
return null;
},
),
const Gap(20),
ElevatedButton(
onPressed: registerAccount,
style: ElevatedButton.styleFrom(
backgroundColor: Colors.amber,
),
child: const Text(
'Register',
style: TextStyle(
color: Colors.white,
fontSize: 20,
),
),
),
const Gap(30),
InkWell(
onTap: navigateToLoginScreen,
child: const Text(
'Already have an account? Click here to login',
),
),
],
),
),
),
),
),
),
),
);
}
}
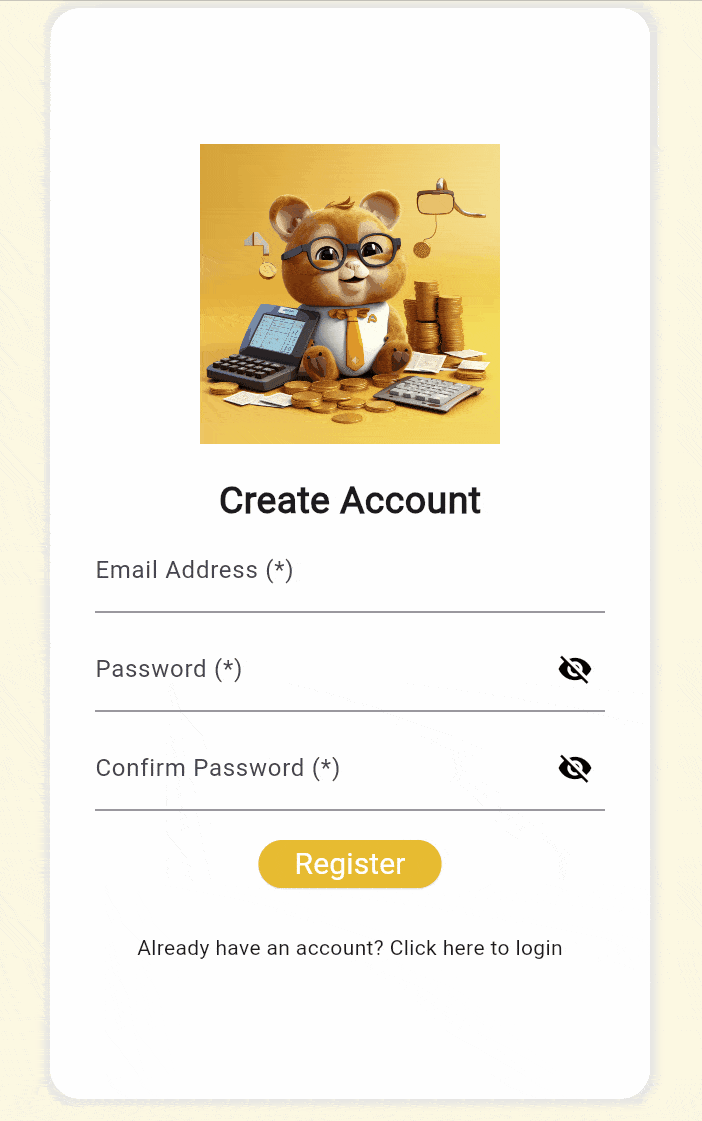