This tutorial demonstrates how to enable geolocation on Android device.
Microsoft Visual Studio Community 2022 (64-bit) - Preview Version 17.1.0 Preview 2.0 Microsoft .NET Framework Version 4.8.09014
1. Let’s add label and button on the main page (MainPage.xaml).
<Label
Text="My Latitude and Longtidue: "
Grid.Row="2"
FontSize="18"
FontAttributes="Bold"
x:Name="LatLonLabel"
HorizontalOptions="Center"
/>
<Button
Text="Get My Location"
FontAttributes="Bold"
Grid.Row="3"
Clicked="GetMyLocationButtonClicked"
HorizontalOptions="Center" />
2. Then add button click listener method in MainPage.xaml.cs.
private async void GetMyLocationButtonClicked(object sender, EventArgs e)
{
try
{
var location = await Geolocation.GetLastKnownLocationAsync();
if(location == null)
{
location = await Geolocation.GetLocationAsync(new GeolocationRequest()
{
DesiredAccuracy = GeolocationAccuracy.High,
Timeout = TimeSpan.FromSeconds(30)
});
}
if(location == null)
{
LatLonLabel.Text = "Something went wrong. Cannot get your location.";
}
else
{
LatLonLabel.Text = $"My Latitude and Longtidue: {location.Latitude}, {location.Longitude}";
}
}catch(Exception ex)
{
Console.WriteLine(ex.Message);
}
}
3. Make sure to add android permission to enable location access of your device in AndroidManifest.xml (Located in Platforms/Android). Put them inside of <manifest>.
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
Done!! Now run the application and check if your devices prompts the location access request and retrieve your location.
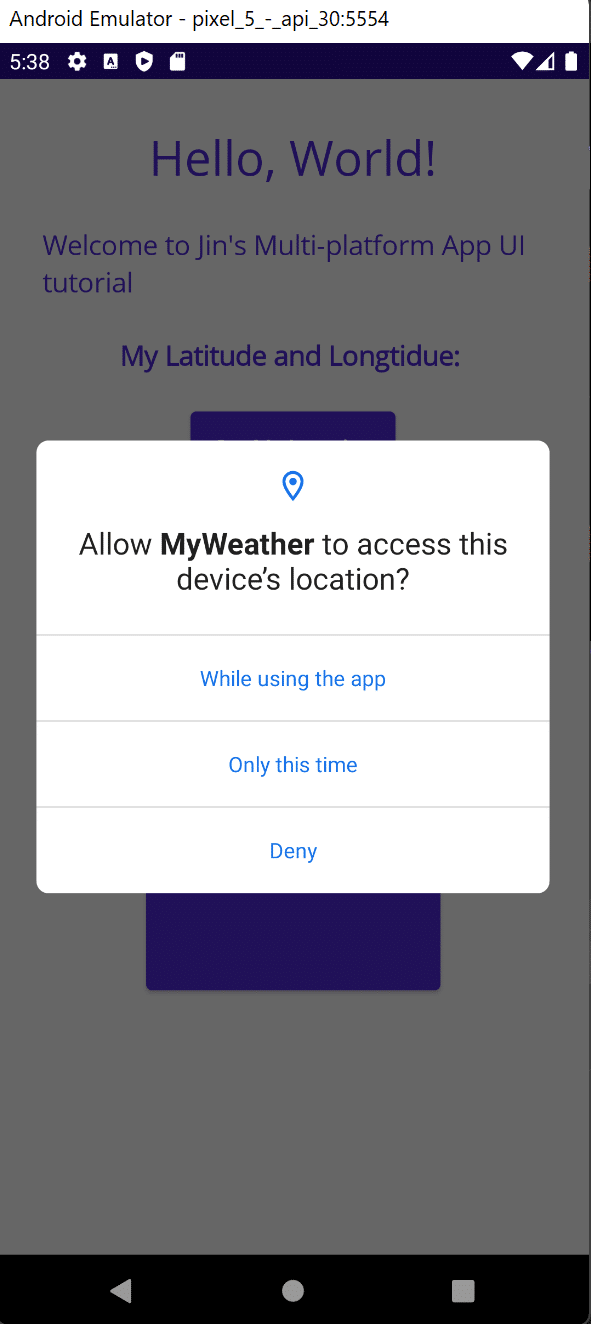
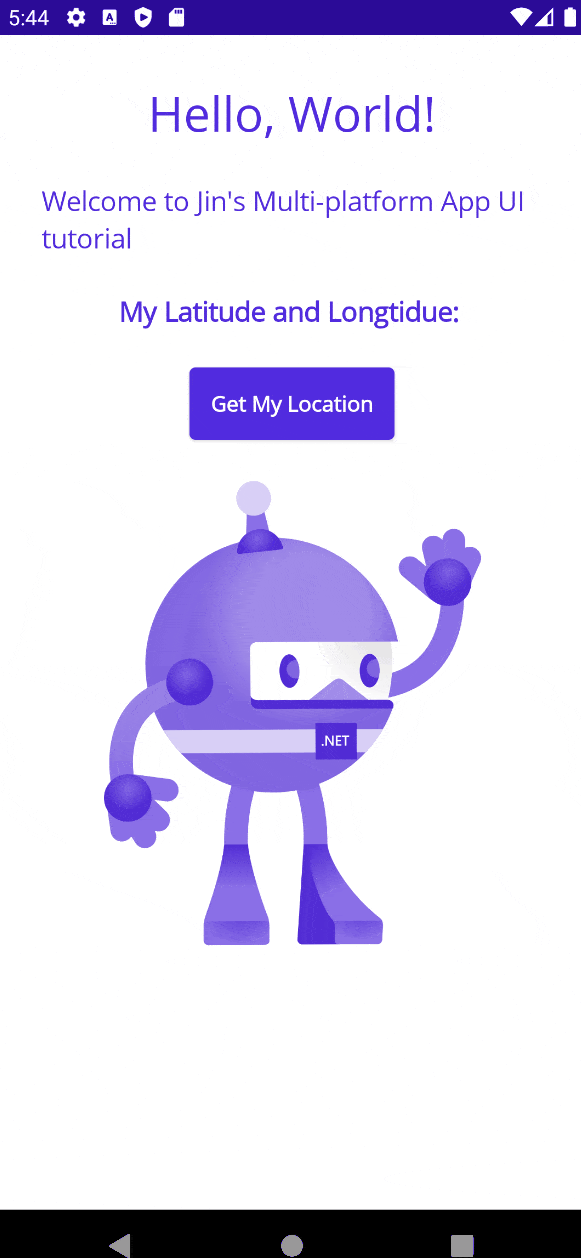